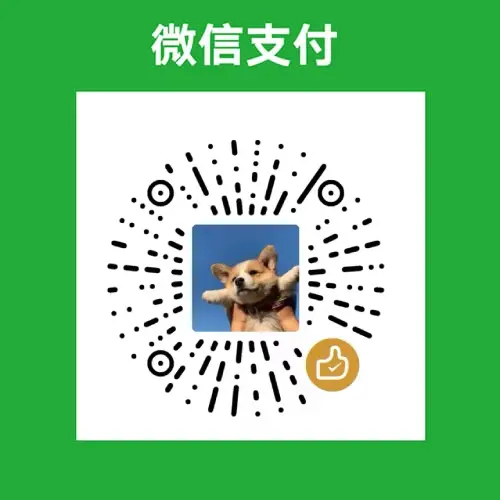
聚合标注
TIP
聚合标注是在不同地图分辨率下,通过聚合方式展现标注点的一种方法。
代码如下:
点我查看代码
vue
<template>
<div id="top">
<el-button type="primary" @click="handleAdd">添加聚合标注</el-button>
<el-button type="danger" @click="handleDelete">移除聚合标注</el-button>
</div>
<div id="map"></div>
</template>
<script lang="ts" setup>
import { onMounted, onBeforeUnmount } from "vue";
import { Map, View } from "ol";
import { Tile as TileLayer } from "ol/layer";
import { defaults, FullScreen } from "ol/control";
import { XYZ } from "ol/source";
import { ATTRIBUTIONS, MAPURL } from "../../../constants";
import { addClusterLabels, removeClusterLabels, clusters } from "./clusters";
let map: Map | null = null;
const raster = new TileLayer({
source: new XYZ({
attributions: ATTRIBUTIONS,
url: MAPURL,
maxZoom: 20,
}),
});
const initMap = () => {
map = new Map({
//初始化map
target: "map",
//地图容器中加载的图层
layers: [
//加载瓦片图层数据
raster,
clusters, // 聚合标注图层
],
view: new View({
center: [0, 0],
minZoom: 2,
zoom: 2,
}),
//加载控件到地图容器中
controls: defaults().extend([
new FullScreen(), //加载全屏显示控件(目前支持非IE内核浏览器)
]),
});
};
// 添加聚合要素
const handleAdd = () => {
if (map) {
addClusterLabels(map);
}
};
// 移除聚合要素
const handleDelete = () => {
if (map) {
removeClusterLabels(map);
}
};
onMounted(() => {
initMap();
if (map) {
removeClusterLabels(map);
}
});
onBeforeUnmount(() => {
if (map) {
map.dispose();
map = null;
}
});
</script>
<!-- Add "scoped" attribute to limit CSS to this component only -->
<style scoped>
#map {
height: 650px;
}
#top {
text-align: center;
height: 50px;
line-height: 50px;
}
</style>
clusters 代码如下:
点我查看代码
ts
import { Feature, Map } from 'ol'
import { Point } from 'ol/geom'
import { Vector as VectorSource, Cluster } from 'ol/source'
import { Vector as VectorLayer } from 'ol/layer'
import { Style, Circle, Stroke, Fill, Text } from 'ol/style'
//此示例创建10000个要素
const count = 10000
const features: Feature<Point>[] = new Array(count)
const e = 4500000
for (let i = 0; i < count; ++i) {
const coordinates = [2 * e * Math.random() - e, 2 * e * Math.random() - e]
features[i] = new Feature(new Point(coordinates))
}
//矢量要素数据源
const source = new VectorSource({
features: features,
})
//聚合标注数据源
const clusterSource = new Cluster({
distance: 40,
source: source,
})
//加载聚合标注的矢量图层
const styleCache = {}
export const clusters = new VectorLayer({
source: clusterSource,
style: (feature): Style[] => {
const size: number = feature.get('features').length
let style: Style[] = styleCache[size]
if (!style) {
style = [
new Style({
image: new Circle({
radius: 10,
stroke: new Stroke({
color: '#fff',
}),
fill: new Fill({
color: '#3399CC',
}),
}),
text: new Text({
text: size.toString(),
fill: new Fill({
color: '#fff',
}),
}),
}),
]
styleCache[size] = style
}
return style
},
})
/**
* 添加聚合要素
* @param {ol.Map} map 地图实例
*/
export const addClusterLabels = (map: Map) => {
//当前聚合标注数据源中的要素
const currentFeatrues = clusterSource?.getSource()?.getFeatures()
//如果聚合标注数据源中没有要素,则重新添加要素
if (currentFeatrues && currentFeatrues.length == 0) {
clusterSource?.getSource()?.addFeatures(features)
clusters.setSource(clusterSource)
map.addLayer(clusters)
}
}
/**
* 移除聚合要素
* @param {ol.Map} map 地图实例
*/
export const removeClusterLabels = (map: Map) => {
//当前聚合标注数据源中的要素
const currentFeatrues = clusterSource?.getSource()?.getFeatures()
//如果聚合标注数据源中没有要素,则重新添加要素
if (currentFeatrues && currentFeatrues.length != 0) {
//移除聚合标注数据源中的所有要素
clusterSource?.getSource()?.clear()
//移除标注图层
map.removeLayer(clusters)
}
}